目標物
ご覧の通り未完全です。解決策が分かり次第更新します。
使用ライブラリ
日付の登録
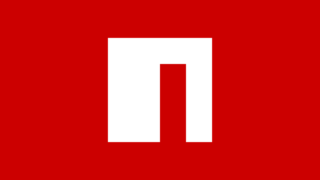
カレンダー
それでは行きましょう!
$ npx create-react-app bigcalendar
$ cd bigcalendar
3つのパッケージを入れます。
$ yarn add react-big-calendar react-datepicker date-fns
$ yarn start
$ npm start
でも開く。どちらでも良い。
編集するファイル
- App.js
- App.css
この2つしか編集しません。
以下のコメントアウト部分を削除する。
logoのimportと、divの中身
// import logo from './logo.svg';
import './App.css';
function App() {
return (
<div className="App">
{/* <header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<p>
Edit <code>src/App.js</code> and save to reload.
</p>
<a
className="App-link"
href="https://reactjs.org"
target="_blank"
rel="noopener noreferrer"
>
Learn React
</a>
</header> */}
</div>
);
}
export default App;
Appクラスだけ残して後は削除する。
.App {
text-align: center;
}
/*
.App-logo {
height: 40vmin;
pointer-events: none;
}
@media (prefers-reduced-motion: no-preference) {
.App-logo {
animation: App-logo-spin infinite 20s linear;
}
}
.App-header {
background-color: #282c34;
min-height: 100vh;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
font-size: calc(10px + 2vmin);
color: white;
}
.App-link {
color: #61dafb;
}
@keyframes App-logo-spin {
from {
transform: rotate(0deg);
}
to {
transform: rotate(360deg);
}
} */
App.jsを編集していく。
①いくつか必要なライブラリをimportする
②localeを設定する
③カレンダーに登録するダミーデータを作成する
④カレンダー内に表示するCalendarコンポーネントを追加する
これら書き換えをしてサーバーを開くと、、、以下のようなエラーが発生
Failed to compile
./src/App.js
Module not found: Can't resolve 'date-fns/format' in 'C:\Users\takag\workspace\react-calender\bigcalendar\src'
”Moduleが無い”
どういうことでしょう。。
こちらの記事をもとに、
$ yarn add date-fns
エラー解消。
次は他のエラー
Module not found: Can't resolve 'react-big-calendar'
次はreact-big-calendarが無いと言われた。。
パッケージのインストールがうまくできていないようなので再度、最初に実行した以下をやってみる。
$ yarn add react-big-calendar react-datepicker date-fns
すると今度は以下のエラー
src\App.js
Line 19:3: 'locales' is not defined no-undef
これは単純にApp.js内で定義しているところのスペルミスだった。
これで、カレンダービューの完成
コードの説明
ここまでのコードをまとめます。
編集ファイルはApp.jsのみ
import './App.css';
import { Calendar, dateFnsLocalizer } from "react-big-calendar";
import format from "date-fns/format";
import ja from "date-fns/locale/ja";
import parse from "date-fns/parse";
import startOfWeek from "date-fns/startOfWeek";
import getDay from "date-fns/getDay";
import "react-big-calendar/lib/css/react-big-calendar.css";
import React, { useState } from "react";
import DatePicker from "react-datepicker";
const locales = {
"ja": require("date-fns/locale/ja")
}
const localizer = dateFnsLocalizer({
format,
parse,
startOfWeek,
getDay,
locales
})
const events = [
{
title: "MTG",
allDay: true,
start: new Date(2021,10,18),
end: new Date(2021,10,18)
},
{
title: "長期休暇",
allDay: true,
start: new Date(2021,10,19),
end: new Date(2021,10,25)
},
{
title: "出張",
allDay: true,
start: new Date(2021,10,26),
end: new Date(2021,10,30)
}
]
function App() {
return (
<div className="App">
<Calendar
localizer = {localizer}
events = {events}
starAccessor="start"
endAccessor="end"
style={{height:500, margin: "50px"}}
/>
</div>
);
}
export default App;
useStateは現時点では使用していないですが後から使います。
一行ずつコードの意味を解説します。
import { Calendar, dateFnsLocalizer } from "react-big-calendar";
react-big-calendarというライブラリから、Calendarコンポーネントと、dateFnsLocalizerコンポーネントをimport
この react-big-calendar は、カレンダーを形作るもの。
import format from "date-fns/format";
date-fnsとは、JavaScriptの日付を扱うライブラリ。
その中の、formatは日付のフォーマットを変更することができる。
import parse from "date-fns/parse";
文字列で入力された日時をDate型に変換する。
import startOfWeek from "date-fns/startOfWeek";
取得した日付の、週の始まり(日曜日)の日時を返す。
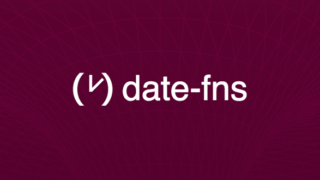
import getDay from "date-fns/getDay";
取得した日が週の何日目かを返す。
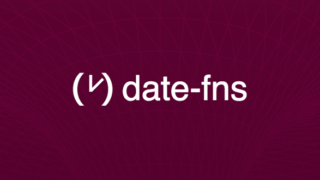
import "react-big-calendar/lib/css/react-big-calendar.css";
Big calenderのデザインを形づくるcssをimport
import React, { useState } from "react";
react から useState コンポーネントをimport
react-datepicker とは、日付登録の際にカレンダーから選択できるライブラリ
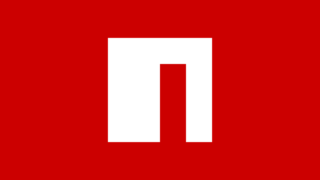
ここから、新規登録ができるように入力フォーム等を追加していく。
newEventとallEventを定義
const [ newEvent, setNewEvent ] = useState({title: "", start: "", end: ""})
const [ allEvents, setAllEvents ] = useState(events)
allEventsとnewEventをまとめ、handleAddEvent という関数で定義する。
「…」は「不特定」を意味する。
function handleAddEvent() {
setAllEvents([...allEvents, newEvent])
}
上で、全て空欄として定義したsetNewEventをnewEventで置き換える処理をする。
<input type="text" placeholder="タイトル" style={{width: "20%", marginRight: "10px"}}
value={newEvent.title} onChange={(e) => setNewEvent({...newEvent, title: e.target.value})}
/>
コメント